how to adjust monitor brightness from command line ?
Hi all, It's been an exciting few months for me. I graduated with a Masters from University of North Carolina at Chapel Hill and moved to Boston in order to work as a Software Engineer at TripAdvisor. In my first week at work, I shifted to a standing desk to avoid sitting for long hours. One of the first challenges I faced while working was how to constantly adjust my monitor brightness so that my eyes don't hurt. I had to lean forward awkwardly (due to the standing desk) to adjust the brightness manually. So, I started digging on how to build a small tool which would allow me to change the monitor brightness from command line and this post describes my findings.
xrandr
All of the google search queries pointed me to a powerful command line tool called xrandr (Xorg RandR) used to configure which display ports are enabled (e.g. LCD, VGA and DVI), and to configure display modes and properties such as orientation, reflection and DPI . xrandr is the command line interface to the RandR X extension. The man pages are your best bet at finding help with xrandr.
Querying the current state of display
First, let's query the current display state of the system. This can be done by running command xrandr -q
.
The current connected display is LVDS1 which has connected written next to it.
Extracting the active display
To extract the name of the active display, you can grep for connected, limit to 1 by piping to head command further piped to cut command and extract the first field.
Change brightness of the active display
We can change the brightness of a display using xrandr by typing the below command.
Here value is a floating point number between 0.0 to 1.0. It's easier to think of the value as a brightness percentage where 1.0 indicates 100% and 0.0 indicates 0%. To change our active display brightness to 85% of it's maximum brightness, we can execute the below command:-
Bash function
We can create a neat little bash function and put it in your ~/.bashrc so that we can execute it on command line.
In the above bash function brightness, we first check if the user passed in the brightness value by checking $# is greater than 0. We set $1 as the brightnessValue (no validation done). Next, we assign the current active display to a variable activeDisplay. If $activeDisplay is not empty, we try executing the xrandr command to change brightness.
Now source your ~/.bashrc file with the command source ~/.bashrc
and you can change the monitor brightness by brightness 0.85
.
This is fine but I need something better to fine-tune brightness
Frankly I was very happy with this command line tool, it was better than awkwardly bending to reach the monitor in order to change brightness, however, I frequently found myself tweaking the brightness between 80-90% (0.80-0.90) and after a couple of adjustments I would settle down.
I had recently worked with PyGTK3 in order to develop an RSI prevention tool for myself called breakmywork. So, I decided to develop a very simple UI with a slider, so that on pressing the left and right arrow keys the brightness should decrease and increase respectively. The GUI should close on pressing escape key.
Extract current brightness
When I first show the slider to the user, I need to set it to current brightness level and we can extract that using below command.
Connecting them all together
Now, all we need to do is put everything together. I won't go into details of the UI building and event handling, however, if you are interested, there is neat documentation at Python GTK+ 3 Tutorial. The code itself is very simple and should be self explanatory.
In your ~/.bashrc you can alias brightness to execute the above python file or alternatively you can create a symlink of the file and place it in /usr/bin.
On executing the command it should launch a neat UI like below and you can control brightness via left/right arrow keys, once done adjusting it, press escape key to quit.
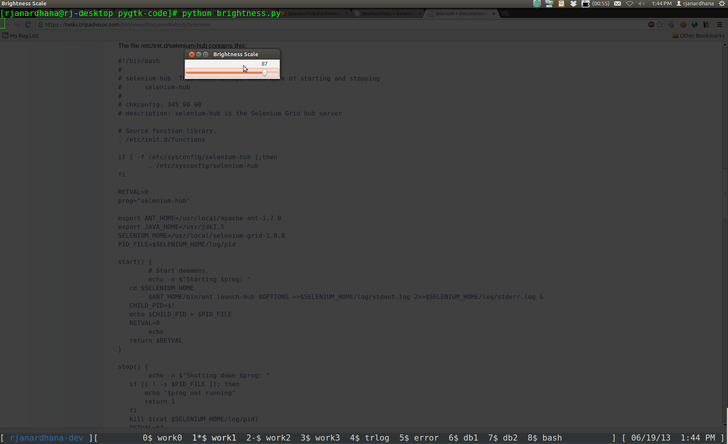
The code can be found on github at https://github.com/ravikiranj/brightness-control. Please note that if you have multiple displays enabled, you will need to run a loop to change the brightness of each one of them.
Comments
Comments powered by Disqus